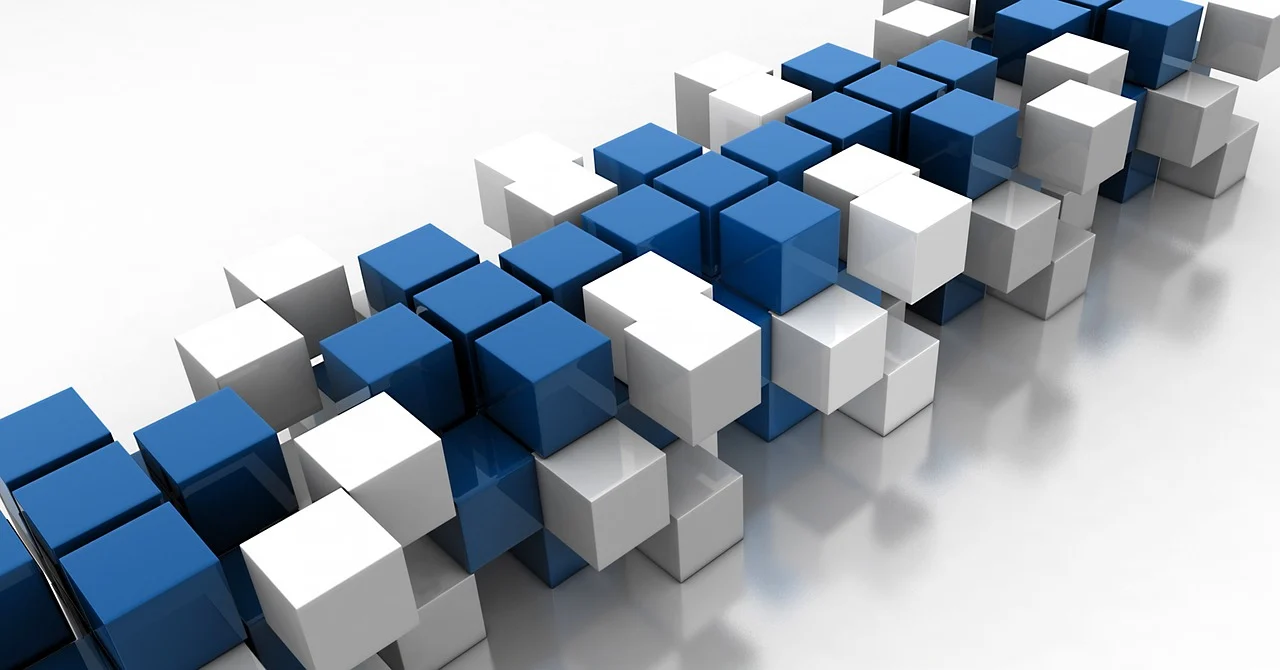
Lombok 使用示例
代码示例:SpringBoot-Labs-Junw jLab-2-1
官方文档:Project Lombok
安装/引用
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
1. @Data
@Data
public class Person {
private String name;
private int age;
}
public class TestData {
// 生成 get、set、toString、equals、hashCode;
@Test
public void testPerson() {
Person person = new Person();
person.setName("John");
person.setAge(30);
assertEquals("Person(name=John, age=30)", person.toString());
}
}
2. @Slf4j
@Slf4j
public class LoggerTest {
/**
* 说明:@Slf4j注释为类生成一个记录器实例,该实例可用于记录消息。
*/
@Test
public void testLogger() {
log.info("Hello, world!");
// Verify that the log message is printed
}
}
3. @Cleanup
public class CleanupTest {
/**
* 使用 @Cleanup 注解处理需要关闭的资源,例如 InputStream、OutputStream、Reader、Writer、Connection 等。
* 将 @Cleanup 注解放置在局部变量声明上,如例所示。
* @throws Exception
*/
@Test
public void testCleanup() throws Exception {
@Cleanup InputStream inputStream = new FileInputStream("src/test/resources/example.txt");
// 使用 inputStream 进行一些操作
byte[] buffer = new byte[1024];
int bytesRead = inputStream.read(buffer);
// 确认读取的数据不为空
assertNotNull(buffer);
// inputStream 会在这里自动关闭
}
}
4. @Builder
@Data
@Builder
public class PersonB {
private String name;
private int age;
}
public class BuilderTest {
/**
* 说明:@Builder注释为 Person 类生成一个构建器类,该类可用于以流畅的 API 样式创建该类的实例。
*/
@Test
public void testBuilder() {
PersonB person = PersonB.builder()
.name("John")
.age(30)
.build();
assertEquals("John", person.getName());
assertEquals(30, person.getAge());
}
}
5. @Accessors
5.1 fluent = true
@Data
@Accessors(fluent = true)
public class PersonC {
private String name;
private int age;
}
public class AccessorsTest {
/**
* fluent
* 默认为false,设置为true时,调用getter、setter方法时,可以省略掉get、set直接[.属性]
*/
@Test
public void testAccessorsFluent() {
PersonC person = new PersonC();
person.name("John");
person.age(30);
assertEquals("John", person.name());
assertEquals(30, person.age());
}
}
5.2 chain = true
@Data
@Accessors(chain = true)
public class PersonD {
private String name;
private int age;
}
public class AccessorsTest {
/**
* chain
* 默认为false,设置为true时,对应字段的 setter 方法调用后,会返回当前对象。
* 且可实现链式编程
*/
@Test
public void testAccessorsChain() {
PersonD person = new PersonD().setName("John").setAge(30);
assertEquals("John", person.getName());
assertEquals(30, person.getAge());
}
}
5.3 prefix = {"xx","yy"}
@Data
@Accessors(prefix = {"xx","yy"})
public class PersonE {
private String xxName;
private int yyAge;
}
public class AccessorsTest {
/**
* prefix
* 该属性是一个字符串数组,当该数组有值时,表示忽略字段中对应的前缀,生成对应的 getter 和 setter 方法。
*/
@Test
public void testPersonPrefix() {
PersonE person = new PersonE();
person.setName("John");
person.setAge(30);
assertEquals("PersonE(xxName=John, yyAge=30)", person.toString());
}
}
6. @NonNull
/**
* @NonNull注释用于指示方法参数或返回值不能为 null。
*/
public class NonNullTest {
@NonNull
public String getNonNullString() {
return "Hello";
}
/**
* 说明: @NonNull注释指示 getNonNullString() 方法返回非 null 值。
*/
@Test
public void testNonNull() {
String nonNullString = getNonNullString();
assertNotNull(nonNullString);
}
}
7. @SneakyThrows
/**
* @SneakyThrows注释用于自动将方法或代码块包装在 try-catch 块中,该块将异常作为运行时异常重新抛出。
*/
public class SneakyThrowsTest {
/**
* 当你想编写抛出检查型异常的代码,但不想在方法签名中声明这些异常时,可以使用 @SneakyThrows。
* 将 @SneakyThrows 注解放置在抛出检查型异常的方法或代码块上。
*/
@SneakyThrows
public void throwException() {
throw new IOException("Example exception");
}
@Test
public void testSneakyThrows() {
try {
throwException();
fail("Expected exception to be thrown");
} catch (RuntimeException e) {
assertEquals("java.io.IOException: Example exception", e.getMessage());
}
}
}
8. @Synchronized
/**
* @Synchronized注释用于自动同步方法或代码块。
*/
public class SynchronizedTest {
private int counter = 0;
@Synchronized
public void incrementCounter() {
counter++;
}
/**
* 说明:@Synchronized注解同步 incrementCounter() 方法,确保一次只能有一个线程执行它。
*/
@Test
public void testSynchronized() {
incrementCounter();
assertEquals(1, counter);
}
}
评论
匿名评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果